This is aimed to be small OpenSCAD Library which re-implements some of its basic forms in discrete manner so vertices remain accessible in order to manipulate before being transformed into actual polyhedron for CSG operations.
State: very experimental state, not yet released due several drawbacks, code released (e.g. polygons must have same amount of segments to properly extrude, resolved)
Updates:
- 2021/01/02: 0.0.6: code finally released
- 2018/12/30: 0.0.5: 3d:
dm_merge([])
merge multiple meshes into single one. - 2018/12/26: 0.0.4:
- 3d:
dm_extrude_rotate(p,n=4,start=0,end=360)
which allows simpledm_torus()
- more detailed documentation and illustrations
- 3d:
- 2018/12/25: 0.0.3:
- 2d: added
dp_translate()
,dp_scale()
,dp_rotate()
,dp_bounds()
anddp_center()
- 3d: added
dm_sphere()
,dm_cylinder()
anddm_cube()
, anddm_translate()
,dm_scale()
,dm_rotate()
,dm_bounds()
anddm_center()
as well
- 2d: added
- 2018/12/24: 0.0.2:
dp_morph()
as part ofdm_extrude()
usesdp_nearest()
to find nearby point of two polygons to morph smoothly, morph examples added - 2018/12/23: 0.0.1: Point Functions, Polygon Functions and Mesh Functions added, code not yet released
Table of Contents
Functions
Discrete Point Functions
dpf_ellipse(r1,r2,p,a=0)
-
dpf_circle(r,p,a=0)
dpf_circle(d,p,a=0)
must used=
diameter
-
dpf_rectangle(w,h,p,a=0)
dpf_square(w,p,a=0)
dpf_npolygon(s,p,a=0)
whereass
amount of sides
each function returns single 2d point, whereas
p
ranges between 0…1, at any resolutiona
is the additional rotate angle (default: 0)
Discrete Polygon Functions
Polygon Functions use Point Functions to create an array/list of 2d points to describe a polygon; n
amount of segments:
Creating Polygons
dp_ellipse(r1,r2,n=12,a=0)
dp_circle(r,n=12,a=0)
dp_circle(d,n=12,a=0)
must used=
diameter- all above use
dpf_ellipse()
to construct the polygon - ellipse and circle is approximated with high count of n-sided polygon
- all above use
dp_rectangle(w,h,n=12,a=0)
dp_square(w,n=12,a=0)
- two above use
dpf_npolygon(sqrt(2)/2,4)
and dp_scale()
if required
- two above use
dp_npolygon(r,s,n=12,a=0,start=0,end=360)
whereass
= amount of sides of the polygon,s
>n
* 2 for good approximation
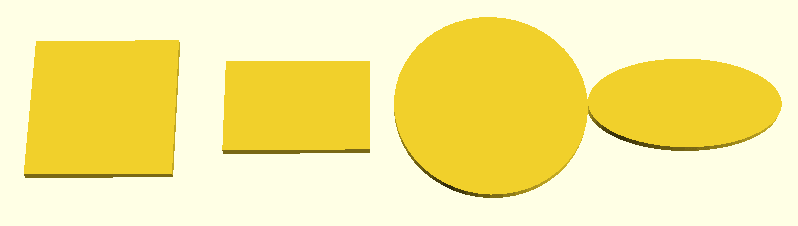

Manipulating Polygons
dp_translate(p,t)
where ast
is a number or array [x,y]dp_scale(p,t)
where ass
is a number or array [x,y]dp_rotate(p,a)
dp_center(p,center=[true,true])
further:
-
dp_subdivide(p,n=2)
n
amount of subdivisions per segment
dp_distort(a,s=1,f=1)
s
amplitude of distortionf
frequency of distortion
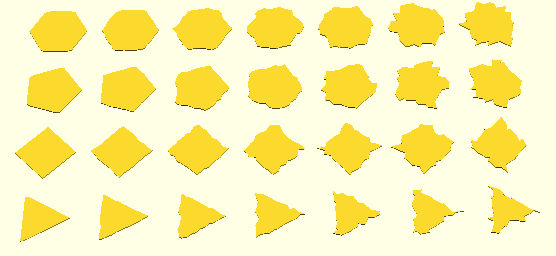
dp_morph(a,b,p)
- morphs between two polygons (
a
andb
), preferably both with same amount of segments and sufficient segments to have smooth transition p
range between 0..1
- morphs between two polygons (
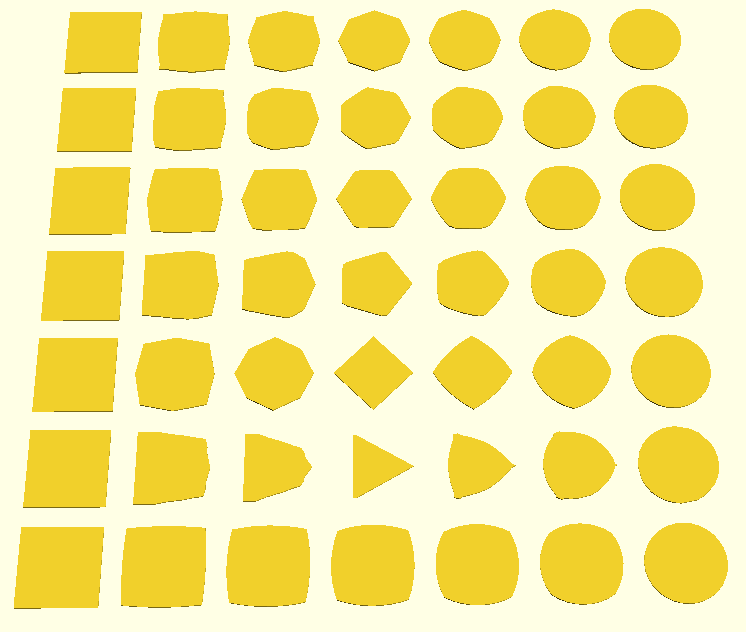
Query Polygons
[[minx,maxx],[miny,maxy]] = dp_bounds(p)
and finally
dp_polygon(p)
- creates an ordinary polygon OpenSCAD object
- Note: you no longer can access the individual vertices
Discrete Mesh Functions
Mesh Functions use Polygon Functions to create an array with two elements:
- list of 3d points
- list of faces using indexes of the 3d points
Creating Meshs
dm_cube(s,center=false)
s
= side length ors
= array of [w,d,h] or [x,y,z]
dm_cylinder(r,h=1,center=false,n=12)
dm_cylinder(r1,r2,h=1,center=false,n=12)
dm_cylinder(d,h=1,center=false)
must used=
diameterdm_cylinder(d1,d2,h=1,center=false,n=12)
must used1=
andd2=
dm_sphere(r,center=false,n=12)
dm_sphere(d,center=false,n=12)
must used=
diameter
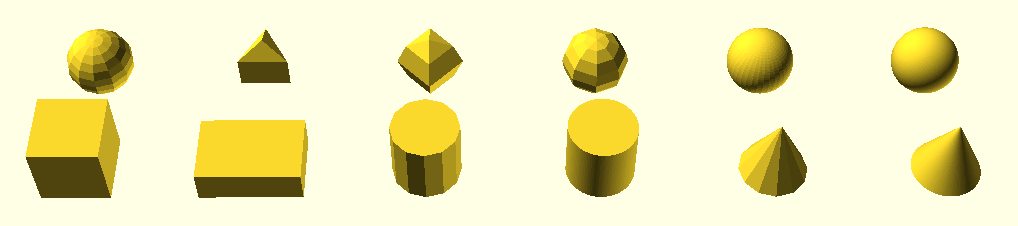
Cube and Cylinder are created by extruding dp_rectangle
respectively dp_circle
, whereas the Sphere is discretely done.
dm_torus(ri=1,ro=4,ni=48,no=48,ai=0,ao=0)
ri
inner radiusro
outer radiusni
andno
inner and outer n-sided polygonai
andao
inner and outer angle offsets
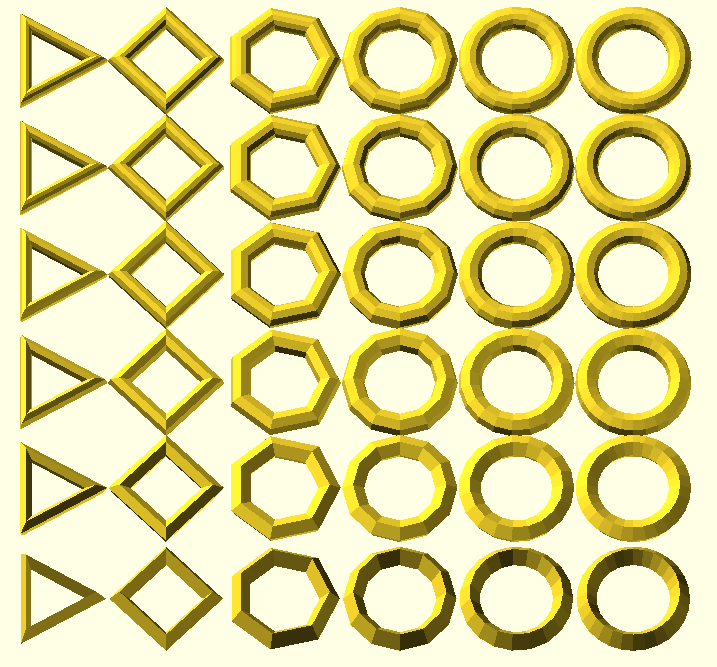
dm_extrude(a,b,s,h)
a
&b
: polygonss
amount of stepsh
height (Z wise)
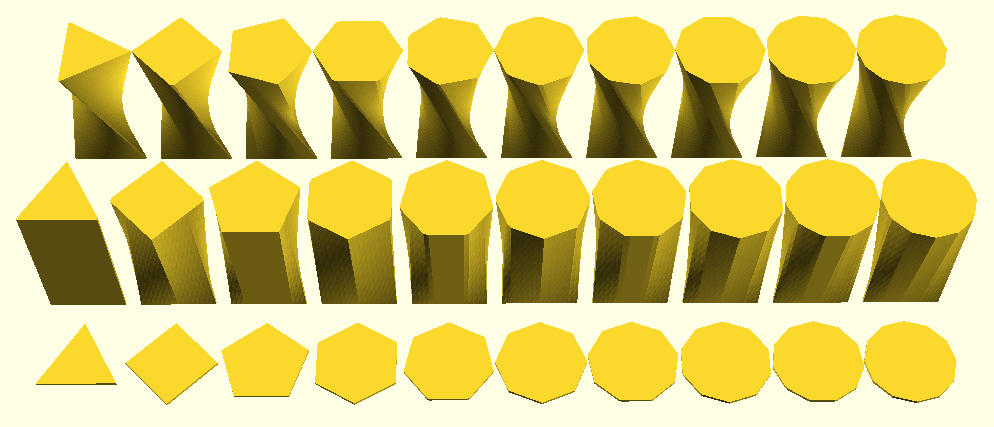
Note: the main aim of this library was to extrude two arbitrary polygons correctly; so dm_extrude()
is the center of this entire undertake.
dm_extrude_rotate(p,n=4,a=0,start=0,end=360)
- sweep or rotate a 2d polygon,
n
-sided, used to implementdm_torus()
- sweep or rotate a 2d polygon,
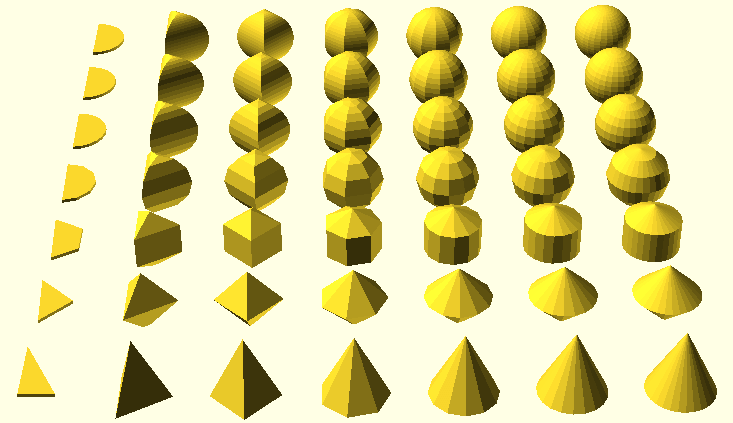
Manipulating Meshs
dm_translate(m,t)
whereast
= number or array with [x,y,z]dm_scale(m,s)
whereass
= number or array with [x,y,z]dm_rotate(m,r)
whereasr
= number or array with [x,y,z]dm_center(m,center=[true,true,true])
dm_merge(ms)
whereasms
is an array of meshes
and more interesting:
dm_distort(m,s=1.0,f=1.0)
- distort the mesh,
s
amplitude,f
frequency
- distort the mesh,
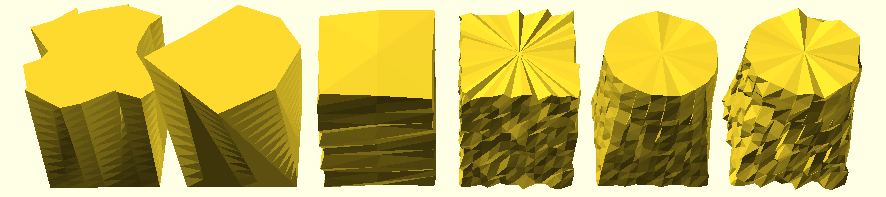
Query Meshs
[[minx,maxx],[miny,maxy],[minz,maxz]] = dm_bounds(m)
and finally
dm_polyhedra(m);
- creates a polyhedra which allows CSG operations
- Note: you no longer can access vertices for manipulation
Todo
- make polygon morph (part of extruding) segment/point count neutral:
usingdp_morph()
which uses dp_nearest(array,point)
to morph between nearest points of polygons- disadvantage: some edges are missed where near-points don’t catch it
- consideration: additional strategies might be required, e.g. if polygon A and B segment count difference is huge, rebalance with using
dp_subdivide()
to achieve this for smoother morphing and extruding - done: walking through
max(len(a),len(b))
through all points and align point indexes linearlya[i*len(a)/max]
andb[i*len(b)/max]
release code, done- extrude along path (e.g.
dm_extrude_along(a,p)
) extrude rotate/sweep (e.g.done:dm_extrude_sweep(a,r)
)dm_extrude_rotate(p,n,start=0,end=360)
more examples, more documentationdone: most functions properly illustrated with examples- implicit meshes: define objects in implicit form (allows more natural objects with smooth edges), and transform it into meshes, see NodeJS Implicit Mesh as reference
- CSG operations on polygon (2d) or mesh (3d) level, quite challenging to do since OpenSCAD are single assignment and
for()
loops must implemented recursively
Download
https://github.com/Spiritdude/DiscreteOpenSCAD
Related Projects
- FunctionalOpenSCAD, alike goal, developed by TheHans
- dotSCAD, innovative library by Justin Lin (JustinSDK)
- Round Anything, CAD rethought for OpenSCAD, starting with points again, by Kurt Hutten